148. 排序链表
题目描述
给你链表的头结点 head
,请将其按 升序 排列并返回 排序后的链表 。
示例 1:
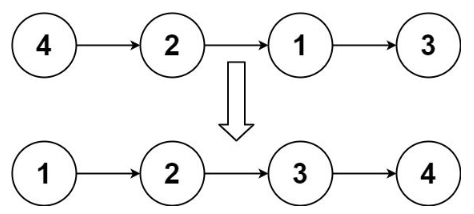
输入:head = [4,2,1,3] 输出:[1,2,3,4]
示例 2:
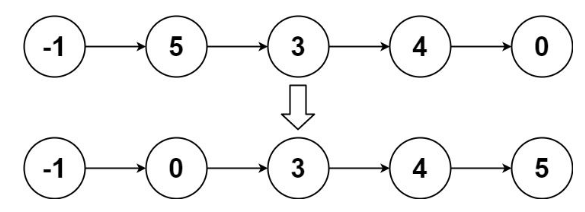
输入:head = [-1,5,3,4,0] 输出:[-1,0,3,4,5]
示例 3:
输入:head = [] 输出:[]
提示:
- 链表中节点的数目在范围
[0, 5 * 104]
内 -105 <= Node.val <= 105
进阶:你可以在 O(n log n)
时间复杂度和常数级空间复杂度下,对链表进行排序吗?
方法一
java
class Solution {
public ListNode sortList(ListNode head) {
if (head == null || head.next == null) {
return head;
}
ListNode slow = head, fast = head.next;
while (fast != null && fast.next != null) {
slow = slow.next;
fast = fast.next.next;
}
ListNode t = slow.next;
slow.next = null;
ListNode l1 = sortList(head);
ListNode l2 = sortList(t);
ListNode dummy = new ListNode();
ListNode cur = dummy;
while (l1 != null && l2 != null) {
if (l1.val <= l2.val) {
cur.next = l1;
l1 = l1.next;
} else {
cur.next = l2;
l2 = l2.next;
}
cur = cur.next;
}
cur.next = l1 == null ? l2 : l1;
return dummy.next;
}
}
cpp
class Solution {
public:
ListNode* sortList(ListNode* head) {
if (!head || !head->next) return head;
auto* slow = head;
auto* fast = head->next;
while (fast && fast->next) {
slow = slow->next;
fast = fast->next->next;
}
auto* t = slow->next;
slow->next = nullptr;
auto* l1 = sortList(head);
auto* l2 = sortList(t);
auto* dummy = new ListNode();
auto* cur = dummy;
while (l1 && l2) {
if (l1->val <= l2->val) {
cur->next = l1;
l1 = l1->next;
} else {
cur->next = l2;
l2 = l2->next;
}
cur = cur->next;
}
cur->next = l1 ? l1 : l2;
return dummy->next;
}
};
ts
function sortList(head: ListNode | null): ListNode | null {
if (head == null || head.next == null) return head;
// 快慢指针定位中点
let slow: ListNode = head,
fast: ListNode = head.next;
while (fast != null && fast.next != null) {
slow = slow.next;
fast = fast.next.next;
}
// 归并排序
let mid: ListNode = slow.next;
slow.next = null;
let l1: ListNode = sortList(head);
let l2: ListNode = sortList(mid);
let dummy: ListNode = new ListNode();
let cur: ListNode = dummy;
while (l1 != null && l2 != null) {
if (l1.val <= l2.val) {
cur.next = l1;
l1 = l1.next;
} else {
cur.next = l2;
l2 = l2.next;
}
cur = cur.next;
}
cur.next = l1 == null ? l2 : l1;
return dummy.next;
}
python
class Solution:
def sortList(self, head: ListNode) -> ListNode:
if head is None or head.next is None:
return head
slow, fast = head, head.next
while fast and fast.next:
slow, fast = slow.next, fast.next.next
t = slow.next
slow.next = None
l1, l2 = self.sortList(head), self.sortList(t)
dummy = ListNode()
cur = dummy
while l1 and l2:
if l1.val <= l2.val:
cur.next = l1
l1 = l1.next
else:
cur.next = l2
l2 = l2.next
cur = cur.next
cur.next = l1 or l2
return dummy.next